DIY Canon RC-1 infrared remote control clone
This article describes how to build a DIY low-budget AVR-based
infrared remote control for Canon cameras.
The reverse-engineered communication protocol of the RC-1 is described
here. The RC-1
lets you trigger the camera instantly or with a delay of 2 seconds
from a distance of up to 5 meters (~16 ft). It comes in very handy
when taking long exposure pictures or for HDR photography.
The presented circuit can be used as part of DIY interval triggers for smaller
Canon cameras without the external trigger connector.
In bracketing mode the RC-1
lets the camera shoot a whole series of images at once!
It is recommended to read the RC-1
communication protocol description first.
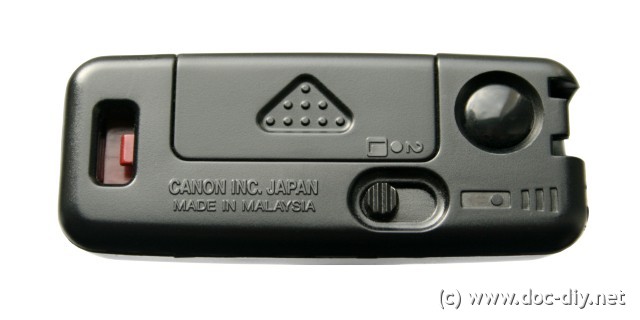
The Circuit
The first circuit I made was based very much on the original Canon (or Mitsumi, see image above) design. It used a crystal oscillator to provide a precise clock to the microcontroller. The IR-LED was driven by an additional NMOS transistor allowing higher current than the controller outputs can source. After I got it working, I simplified the schematics successively to reduce the part count and make it more easy to build. The schematics of the initial "high-end" version is here. The simplified version is shown in the picture below.
I was able to trigger the Canon EOS 400D from more than 5 meters (~16 ft) with both versions. The simple version had slightly more failed trigger attempts. I recommend to build the simplified version though.
In the simplified version the crystal oscillator was replaced by the internal 8 MHz oscillator, saving three external parts. The disadvantage of this solution is that the frequency is drifting slightly with the supply voltage and temperature. Details on this behaviour can be found in the "Electrical Characteristics" section of the Attiny25 data sheet. The most radical simplification was the elimination of the transistor. I found out experimentally that driving the IR-LED directly from paralleled microcontroller pins is powerful enough to trigger the camera from about 5 meters (~16 ft). Finally the voltage reducing diode has been dropped increasing the supply voltage to the "absolute maximum" value of 6 V. It seams to be no problem for the ATtiny25. I don't recommend to leave the mode selector input pin unconnected in the simplified version. I had some sporadic shots in the wrong mode.
AVR source code
The program (simplified version) was written in C and compiled with the avr-gcc compiler in the AVR-Studio. The _delay_ms function was used to generate the delays. After applying power to the controller, pin PB0 is tested for its level. If the level is high (pin not connected, internal pull-up active), the instant trigger signal is emitted. If the pin is tight to ground, the delayed trigger signal is send. After sending the pulses, the microcontroller is put in power-down mode to reduce the power consumption to the minimum. When the pushbutton is released, no current is drawn from the battery, except the leakage current of the capacitor. A high quality cap should be used to keep this current low. The LED output signal is present on pins PB1 and PB2. The pins should be used in parallel if driving the LED directly as in the simplified circuit version. For some inexplicable reasons I wasn't able to parallel more than two pins. Before compilation, the microcontroller clock frequency must be specified in the c-file header according to the oscillator used. If the internal 8 MHz oscillator is used as clock source, an F_CPU of 7.9 MHz is a good choice. The reason for the difference is the voltage drift of the internal RC-oscillator. The nominal frequency of 8 MHz is specified for a supply voltage of 3 V. It's decreasing slightly for higher supply voltages. The dependence is documented in a diagram it the data sheet of the controller.
#define F_CPU 7900000 // clock frequency, set according to clock used! #include <inttypes.h> #include <stdlib.h> #include <avr/io.h> #include <avr/sleep.h> #include <util/delay.h> #include <avr/interrupt.h> #define HPERIOD 0.01524 #define RATIO 0.4 #define NPULSES 16 #define LEDOFF 0b00000001 #define LEDON 0b00010111 int main(void) { uint8_t i; DDRB = 0b00010110; // pin PB0 is input, pins PB1-PB4 are output PORTB = 0b00000001; // pull-up for input pin PB0 asm volatile ("nop"); asm volatile ("nop"); if ( PINB & (1<<PINB0) ) { for(i=0;i<NPULSES;i++) { PORTB = LEDON; _delay_ms(HPERIOD); PORTB = LEDOFF; _delay_ms(HPERIOD); } _delay_ms(7.33); // instant for(i=0;i<NPULSES;i++) { PORTB = LEDON; _delay_ms(HPERIOD); PORTB = LEDOFF; _delay_ms(HPERIOD); } } else { for(i=0;i<NPULSES;i++) { PORTB = LEDON; _delay_ms(HPERIOD); PORTB = LEDOFF; _delay_ms(HPERIOD); } _delay_ms(5.36); // delayed for(i=0;i<NPULSES;i++) { PORTB = LEDON; _delay_ms(HPERIOD); PORTB = LEDOFF; _delay_ms(HPERIOD); } } set_sleep_mode(SLEEP_MODE_PWR_DOWN); sleep_mode(); }
The C source code and the hex-file for the simplified version can be downloaded here
C source code | canon_remote.c |
hex file | canon_remote.hex |
Fuses
I used PonyProg to copy the hex-file into the controller and set the fuses. The fuse settings can be copied from this screenshot
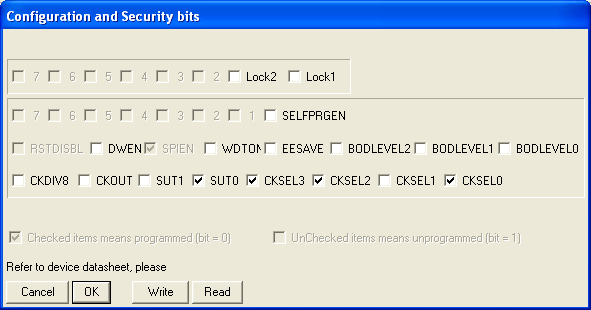
By the way, most digital cameras are sensitive to infrared light in the preview mode, so you can see IR-diodes blinking on the display. This might be helpful for basic debugging of the remote control circuit.
Debugging
Your RC-1 clone doesn't work?
- Check, if any signal is present at the IR LED pins. Use a loudspeaker if no scope at hand.
- In case you can't hear anything probably the programming went wrong or the clock source is not correct in the AVR.
- If the signal is audible, use a video camera or a simple digital camera (mobile phone?) to check the IR signal. Cheap photo equipment is usually sensitive to IR light. In total darkness you can usually see IR LEDs glowing with the naked eye. Do not look directly into the beam! Is the polarity of the IR LED ok? An IR LED and an IR photodiode are two different things.
- In case the IR light is visible and the camera not responding: Check camera settings, the remote/self-timer option must be on! If ok, check the program timing. The _delay_us() routines in the code need a proper setting of the F_CPU variable. A sound card can be used instead of a scope to analyse the signal. Check the max input voltage before!
Links
http://www.doc-diy.net/photo/rc-1_hacked
Canon RC-1 IR remote control reverse-engineered
http://www.atmel.com/dyn/resources/prod_documents/doc2586.pdf
Attiny25 data sheet
http://www.lancos.com/prog.html
PonyProg, my favourite AVR programming tool
http://www.bigmike.it/ircontrol/
A similar AVR based project for Nikon cameras
Disposable Email Temporary Email Temporary Email
the code for the tiny25 will work fine with the tiny45 and tiny85.
Luk
Nearly 60 Minutes of work/testing. It has a range of > 15 meters (lenght ob backyard) or from one room to the other (IR bounced on the walls through the doors).
Yeah, call it overkill, but it works. btw. it is sunday, no chance to buy a canon rc-1 ;)